123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566676869707172737475767778798081828384 |
- name: Validate new issue
- on:
- issues:
- types: ["opened"]
- jobs:
- validate-new-issue:
- runs-on: ubuntu-latest
- steps:
- - uses: actions/checkout@v2
- - name: "Validate new issue"
- shell: bash
- env:
- GITHUB_TOKEN: ${{ github.token }}
- run: |
- issue_number=${{ github.event.issue.number }}
- echo "Validating issue #${issue_number}."
- # Trust users who belong to the getsentry org.
- if gh api "https://api.github.com/orgs/getsentry/members/${{ github.actor }}" >/dev/null 2>&1; then
- echo "Skipping validation, because ${{ github.actor }} is a member of the getsentry org."
- exit 0
- else
- echo "${{ github.actor }} is not a member of the getsentry org. 🧐"
- fi
- # Prep reasons for error message comment.
- REASON="your issue does not properly use one of this repo's available issue templates"
- REASON_EXACT_MATCH="you created an issue from a template without filling in anything"
- REASON_EMPTY="you created an empty issue"
- # Definition of valid:
- # - not empty (ignoring whitespace)
- # - matches a template
- # - all the headings are also in this issue
- # - extra headings in the issue are fine
- # - order doesn't matter
- # - case-sensitive tho
- # - not an *exact* match for a template (ignoring whitespace)
- function extract-headings { { sed 's/\r$//' "$1" | grep '^#' || echo -n ''; } | sort; }
- jq -r .issue.body "$GITHUB_EVENT_PATH" > issue
- if ! grep -q '[^[:space:]]' issue; then
- REASON="${REASON_EMPTY}"
- else
- extract-headings <(cat issue) > headings-in-issue
- for template in $(ls .github/ISSUE_TEMPLATE/*.md 2> /dev/null); do
- # Strip front matter. https://stackoverflow.com/a/29292490/14946704
- sed -i'' '1{/^---$/!q;};1,/^---$/d' "$template"
- extract-headings "$template" > headings-in-template
- echo -n "$(basename $template)? "
- if [ ! -s headings-in-template ]; then
- echo "No headers in template. 🤷"
- elif [ -z "$(comm -23 headings-in-template headings-in-issue)" ]; then
- echo "Match! 👍 💃"
- if diff -Bw "$template" issue > /dev/null; then
- echo "... like, an /exact/ match. 😖"
- REASON="${REASON_EXACT_MATCH}"
- break
- else
- gh api "/repos/:owner/:repo/issues/${issue_number}/labels" \
- -X POST \
- --input <(echo '{"labels":["Status: Unrouted"]}')
- exit 0
- fi
- else
- echo "No match. 👎"
- fi
- done
- fi
- # Failed validation! Close the issue with a comment.
- cat << EOF > comment
- Sorry, friend. As far as this ol' bot can tell, ${REASON}. Please [try again](https://github.com/${{ github.repository }}/issues/new/choose), if you like. (And if I'm confused, please [let us know](https://github.com/getsentry/.github/issues/new?title=template+enforcer+is+confused&body=${{ github.event.issue.html_url }}). 😬)
- ----
- [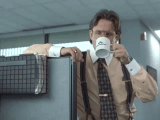](https://www.youtube.com/watch?v=Fy3rjQGc6lA)
- ([log](https://github.com/${{ github.repository }}/actions/runs/${{ github.run_id }}))
- EOF
- echo -n "Commented: "
- gh issue comment ${{ github.event.issue.number }} --body "$(cat comment)"
- gh issue close ${{ github.event.issue.number }}
- echo "Closed with: \"${REASON}.\""
|